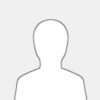
Yazar:
Mak@le
Öncelikle pictureboxun AllowDrop özelliğini True yapın.
PictureBox MouseMove eventine alttaki kodları ekleyin
PictureBox Drag Enter kısmına alttaki kodları ekleyin
PictureBox Drag Drop kısmına alttaki kodları ekleyin.
Kod:
this.pictureBox2.AllowDrop = true;
PictureBox MouseMove eventine alttaki kodları ekleyin
Kod:
private void pictureBox1_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
pictureBox1.DoDragDrop( pictureBox1.Image, DragDropEffects.All );
}
Kod:
private void pictureBox2_DragEnter(object sender, System.Windows.Forms.DragEventArgs e)
{
if ( e.Data.GetDataPresent( DataFormats.Bitmap ) )
{
e.Effect = DragDropEffects.Copy;
}
else
e.Effect = DragDropEffects.None;
}
Kod:
private void pictureBox2_DragDrop(object sender, System.Windows.Forms.DragEventArgs e)
{
if ( (e.Data.GetDataPresent(DataFormats.Bitmap)))
{
this.pictureBox1.Image = (Bitmap)(e.Data.GetData(DataFormats.Bitmap));
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
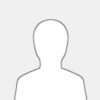
Yazar:
Mak@le
Kod:
private void dataGridView1_MouseDown(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Right & dataGridView1.RowCount>0)
{
var hti = dataGridView1.HitTest(e.X, e.Y);
dataGridView1.ClearSelection();
dataGridView1.Rows[hti.RowIndex].Selected = true;
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
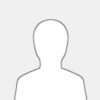
Yazar:
Mak@le
Kod:
using System;
using System.Data;
using System.Windows.Forms;
using ClosedXML.Excel;
namespace Proje1
{
class xlsxAktar
{
public static void xlsxKaydet(DataGridView veriTablosu)
{
try
{
DataTable tablo_ = new DataTable();
foreach (DataGridViewColumn sutun in veriTablosu.Columns)
{
tablo_.Columns.Add(sutun.HeaderText, sutun.ValueType);
}
foreach (DataGridViewRow satir in veriTablosu.Rows)
{
tablo_.Rows.Add();
foreach (DataGridViewCell hucre in satir.Cells)
{
tablo_.Rows[tablo_.Rows.Count - 1][hucre.ColumnIndex] = hucre.Value.ToString();
}
}
SaveFileDialog dosyakaydet = new SaveFileDialog();
dosyakaydet.FileName = "xlsxProjeDosyaAdı"; // burada dosya adı belirtilebilir
dosyakaydet.InitialDirectory = Environment.SpecialFolder.Desktop.ToString();
dosyakaydet.Filter = "XLSX Office Dosyası|*.xlsx"; // Dosya uzantısı burada belirtilebilir.
if (dosyakaydet.ShowDialog() == DialogResult.OK)
{
using (XLWorkbook calismaKitabi = new XLWorkbook())
{
calismaKitabi.Worksheets.Add(tablo_, "Proje1");
calismaKitabi.SaveAs(dosyakaydet.FileName);
MessageBox.Show("Office Exel XLSX dosyası başarıyla oluşturuldu!\n" + "Dosya Konumu: " + dosyakaydet.FileName, "İşlem Tamam");
}
}
}
catch (Exception hata)
{
MessageBox.Show(hata.Message);
}
}
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
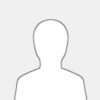
Yazar:
Mak@le
Kod:
using System.IO;
using System.Windows.Forms;
using System;
namespace Proje1
{
class txtAktar
{
public static void txtKaydet(DataGridView veriTablosu)
{
try
{
SaveFileDialog dosyakaydet = new SaveFileDialog();
dosyakaydet.FileName = "projeTxtDosyaAdı";
dosyakaydet.InitialDirectory = Environment.SpecialFolder.Desktop.ToString();
dosyakaydet.Filter = "Txt Dosyası|*.txt";
if (dosyakaydet.ShowDialog() == DialogResult.OK)
{
TextWriter txt = new StreamWriter(dosyakaydet.FileName);
foreach (DataGridViewColumn sutun in veriTablosu.Columns)
{
txt.Write(sutun.HeaderText + " ");
}
txt.Write("\n");
foreach (DataGridViewRow satir in veriTablosu.Rows)
{
foreach (DataGridViewCell hucre in satir.Cells)
{
txt.Write(hucre.Value.ToString() + " ");
}
txt.Write("\n");
}
txt.Close();
MessageBox.Show("TXT dosyası başarıyla oluşturuldu!\n" + "Dosya Konumu: " + dosyakaydet.FileName, "İşlem Tamam");
}
}
catch(Exception hata)
{
MessageBox.Show(hata.Message);
}
}
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
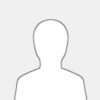
Yazar:
Mak@le
Kod:
private string SizeOne(String s)
{
if(s.Length>1)
{
return s[0].ToString().ToUpper() + s.Substring(1);
}
else
{
return s;
}
}
Kodu formda boş bir yere ekleyin. Kullanımı oldukça basit.
textBox1.Text = SizeOne(textBox1.Text);
veya
textBox1.Text = SizeOne("avrupa");
Forum:
C# (Csharp)
Yorum
Yorum Yok
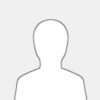
Yazar:
Mak@le
Kod:
using System;
using System.IO;
using System.Windows.Forms;
using iTextSharp.text.pdf;
using iTextSharp.text;
namespace Proje1
{
class pdfAktar
{
public static void pdfKaydet(DataGridView veriTablosu)
{
try
{
PdfPTable pdfTablosu = new PdfPTable(veriTablosu.ColumnCount);
pdfTablosu.DefaultCell.Padding = 3;
pdfTablosu.WidthPercentage = 100;
pdfTablosu.HorizontalAlignment = Element.ALIGN_LEFT;
pdfTablosu.DefaultCell.BorderWidth = 1;
foreach (DataGridViewColumn sutun in veriTablosu.Columns)
{
PdfPCell pdfHucresi = new PdfPCell(new Phrase(sutun.HeaderText));
pdfHucresi.BackgroundColor = Color.LIGHT_GRAY;
pdfTablosu.AddCell(pdfHucresi);
}
foreach (DataGridViewRow satir in veriTablosu.Rows)
{
foreach (DataGridViewCell cell in satir.Cells)
{
pdfTablosu.AddCell(cell.Value.ToString());
}
}
SaveFileDialog dosyakaydet = new SaveFileDialog();
dosyakaydet.FileName = "projePDfDosyaAdı";
dosyakaydet.InitialDirectory = Environment.SpecialFolder.Desktop.ToString();
dosyakaydet.Filter = "PDF Dosyası|*.pdf";
if (dosyakaydet.ShowDialog() == DialogResult.OK)
{
using (FileStream stream = new FileStream(dosyakaydet.FileName, FileMode.Create))
{
Document pdfDoc = new Document(PageSize.A4, 10f, 10f, 10f, 0f);
PdfWriter.GetInstance(pdfDoc, stream);
pdfDoc.Open();
pdfDoc.Add(pdfTablosu);
pdfDoc.Close();
stream.Close();
MessageBox.Show("PDF dosyası başarıyla oluşturuldu!\n" + "Dosya Konumu: " + dosyakaydet.FileName, "İşlem Tamam");
}
}
}
catch (Exception hata)
{
MessageBox.Show(hata.Message);
}
}
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
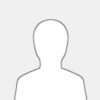
Yazar:
Mak@le
İlk olarak en üst kısma bu kütüphaneleri import ediyoruz
using System.Drawing;
using System.Drawing.Imaging;
Daha sonra 1 button ekleyelim ve Click eventine şu kod satırlarını ekleyelim.
Bitmap bitmap = new Bitmap(button1.Width,button1.Height, button1.CreateGraphics());
button1.DrawToBitmap(bitmap,new Rectangle(0,0,bitmap.Width,bitmap.Height));
pictureBox1.Image = bitmap;
bitmap.Save("c://screenshot.png",ImageFormat.Png);
Yukarıda ki örnekte gördüğünüz üzere Button1'in ekran görüntüsünü alıyoruz. Bu kod ile thumbnial maker gibi yazılımlar yapabilirsiniz. Button yerine panel veya picturebox kullanabilirsiniz.
using System.Drawing;
using System.Drawing.Imaging;
Daha sonra 1 button ekleyelim ve Click eventine şu kod satırlarını ekleyelim.
Bitmap bitmap = new Bitmap(button1.Width,button1.Height, button1.CreateGraphics());
button1.DrawToBitmap(bitmap,new Rectangle(0,0,bitmap.Width,bitmap.Height));
pictureBox1.Image = bitmap;
bitmap.Save("c://screenshot.png",ImageFormat.Png);
Yukarıda ki örnekte gördüğünüz üzere Button1'in ekran görüntüsünü alıyoruz. Bu kod ile thumbnial maker gibi yazılımlar yapabilirsiniz. Button yerine panel veya picturebox kullanabilirsiniz.
Forum:
C# (Csharp)
Yorum
Yorum Yok
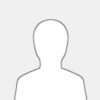
Yazar:
Mak@le
Alıntı:Kod:comboBox1.Items.Clear();
String[] Sehirler = {"Ankara","İzmir","İstanbul","Trabzon"};
//comboBox1.Items.AddRange(new[]{"Ankara","İzmir","İstanbul","Trabzon"});
comboBox1.Items.AddRange(Sehirler);
comboBox1.SelectedIndex = 0;
Forum:
C# (Csharp)
Yorum
Yorum Yok
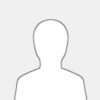
Yazar:
Mak@le
Alıntı: private void btnKaydet_Click(object sender, EventArgs e)
{
SaveFileDialog dosyakaydet = new SaveFileDialog();
if (dosyakaydet.ShowDialog() == DialogResult.OK)
{
System.IO.File.WriteAllBytes(dosyakaydet.FileName, ResProje.Properties.Resources.resFile);
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
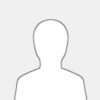
Yazar:
Mak@le
Alıntı:Kod:using System;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
namespace DizinBulYazdir
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
string seciliDizin;
private void button1_Click(object sender, EventArgs e)
{
try
{
if (textBox1.Text == string.Empty)
{
MessageBox.Show("Bulunacak metin girin");
return;
}
FolderBrowserDialog dizinsec = new FolderBrowserDialog();
if (dizinsec.ShowDialog() == DialogResult.OK)
{
seciliDizin = dizinsec.SelectedPath;
}
string[] bul = Directory.GetDirectories(seciliDizin, textBox1.Text, SearchOption.AllDirectories);
// string [] bul = Directory.GetFiles(seciliDizin, textBox1.Text, SearchOption.AllDirectories);
for (int i = 0; i < bul.Length; i++)
{
if (bul[i] != null)
{
richTextBox1.AppendText(bul[i] + "r\n\r\n");
}
else
{
break;
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
}
Forum:
C# (Csharp)
Yorum
Yorum Yok
Hoşgeldin, Ziyaretçi
Forumda Ara
Forum İstatistikleri
Son Yazılanlar
Web Sitenizin Trafiğini A...
Son Yorum:
ofelya
•
07.07.2024 17:34
Twitter Account Generator...
Son Yorum:
ofelya
•
24.06.2024 12:15
Discord Sunucumuza Katılı...
Son Yorum:
ofelya
•
29.05.2024 11:04
Discord Oto. Mesaj Zamanl...
Son Yorum:
ofelya
•
20.04.2024 01:59
C# Hosts Dosayasını Düzen...
Son Yorum:
redzii
•
19.03.2024 00:05
C# Astape UI - WPF UI Des...
Son Yorum:
admin
•
29.12.2023 10:56
Forum Sitemiz Xenforo'ya ...
Son Yorum:
admin
•
11.12.2023 20:32
C# FatalityHack Loader Ui...
Son Yorum:
admin
•
11.12.2023 15:55
C# ile Veritabanına Bağla...
Son Yorum:
admin
•
25.11.2023 20:08